Operators
int a = 3;
int b = 4;
int c = a + b; // c = 7
int d = 2 * a - b; // d = 2
int e = c / d; // e = 3
- If the result of dividing two integers is an integer, return that result
- If the result of dividing two integers is a rational, return the closest integer smaller than the rational
- Pre-increment and pre-decrement(++var and –var)
- Post-increment and post-decrement(var++ and var–)
int a = 3;
int b = a++; // b = 3 and a = 4
int c = --a; // a is firstly decremented; a = 3, then its assigned to c; c = 3
int d = --a + b++ + c--; /* a = 2; b's and c's old values are used while calculating d
because of the post increment/decrement (b = 3; c = 3;). d = 8; After the value is stored in d,
b and c are incremented, decremented respectively*/
Finally, there are special type of operations which are a combination of arithmetic operators and the assignment operator. The functionality of those operators is to do the supplied operation with the old value of the variable on the left and the expression evaluated on the right and to assign the newly formed result to the variable on the left:
int a = 1;
int b = 2;
int b += a; // Equivalent to b = b + a; b = 3
a -= a - b; // a = a - (a - b); a = 3
int c = 6;
c /= a + b; // c = c / (a + b); c = 1
c *= c + a * b; // c = c * (c + a * b); c = 10
One additional operation you have when dealing with integers is the modulo (denoted as “%“). The result of this operation represents the remainder of division.
int a = 38;
int b = a % 3; // 38 / 3 = 12 with remainder 2. So b = 2
int x = 3;
int y = x % 2; // 3 / 2 = 1 with remainder 1. So y = 1
a %= (x+y)*b; // Equivalent to a = a % ((x + y) * b); a = 6
When it comes to floating point numbers, everything is the same as in math. Here we don’t have a problem with division because dividing two rational numbers is bound to give us a rational, which can be represented by a floating point data type.
float x = 3.1;
float y = 2.0;
float a = x + y; // a = 5.1
a = x * y; // a = 6.2
a = 2 * x / y; // a = 3.1
Finally, one of the most useful operators you might use when doing any complicated calculation is the bracket operator. It works the same way as in math. Its functionality is to group the order of operations which are being evaluated in your expression.
int a = 2 + 5 * 3; // 17
int b = (2 + 5) * 3; // 21
Binary
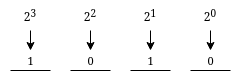
- At the top of the left column, put the number you want to represent in the binary system (10 in our case)
- Calculate the modulo of that number with 2 and put it next to it in the right column
- Calculate the integer quotient (whole division) of that number with 2 and put it below it in the same column (left)
- Repeat the process for every row until you get 1 in the left column
- The binary number is read from the right column, from the bottom to the top
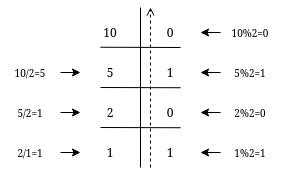
Binary logical operation
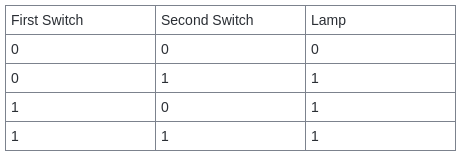
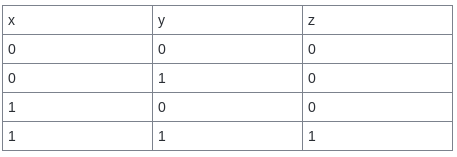
Logical and bitwise operators operators in C++
- Logical operators
- Bitwise operators
- And operator – represented as “&&“. If both operands are true, it returns true.
- Or operator – represented as “||“. If either operand is true, it returns true.
- Equals operator – represented as “==“. If both operands have the same value, it returns true. This works for any primitive data type
- Not equals operator – represented as “!=“. Has the opposite functionality of the equals operator
bool first_condition = (true && false) == (false || true); // You figure it out Einstein
bool last_condition = first_condition != true;
- The pair of variables get transformed into their binary representation
- Next, each pair of bits starting from the right of each variable is used in the operation
- The bit operation gets evaluated and stored in the result in the same position as the position of the bits in the pair of variables.
- Every pair of bits then gets evaluated in the same fashion and the resulting value gets transformed from its binary representation into the adequate data type.
int x = 0b1011; //Binary representation of the decimal number 11 assigned to an integer
int y = x + 0b01; /* y is assigned the value x plus the binary representation of the number 1;
y = 12;*/
- And (&)
- Or (|)
- Shift left, shift right (<< and >>)
- Xor (^)
int x = 0b0001;
int y = 0b0010;
int c = x & y; // c = 0b0000
int d = x | y; // d = 0b0011
Shifting represents an operation of moving the bits to the left or to the right of the first operand by a value defined as the second operand. The whole process is actually multiplying the first operand by a power of 2 defined by the second operand (shift left) or dividing the first operand by a power of 2 defined by the second operand (shift right).
int x = 3;
int y = x << 2; // y = 12
int z = y >> 1; // z = 6
Lastly, there is the xor operation. It is similar to and and or, but has a different way of working with the bits. The xor operation takes each pair of bits and if they are of the same value, gives the result 0. If the bits are different, it gives the result 1.
int a = 0b011; //a = 3
int b = 0b101; //b = 5
int x = a ^ b; // x = 0b110; x = 6
Table of contents
- Loops, Pointers and Functions
- Classes and Objects
- Operator Overloading
- Class Inheritance
- Exceptions
- Namespaces
- Templates
- Standard Library
- Additional Problems