Python Introduction
Python as a programming language
Python environment
A lot of Python environments have an interactive mode, which is good for people who are new in Python. That means we can test a line of code by writing it and the output(s) is(are) immediately displayed. Here’s a few examples:
>>> 3 + 4
7
Every new line begins with >>>, which means we can start writing our code. Then we write the command 3 + 4 and after tapping ENTER on the keyboard we immediately get the output, which is 7. As we can see, output line doesn’t begin with >>> and we cannot change it.
>>> print("Wipher is good")
Wipher is good
Now we want to write a program that will show us a message on the output. We can do that using the function print and inside its brackets ( () ) writing a message we want under quotation marks ( ” ” ). As a result, we get the message on the output which says Wipher is good. Is it not?
>>> x = 12
>>> print(x)
12
There is one more example of how we can use the print function, but this time it prints the value of previously defined variable x, because we didn’t use quotation marks ( ” “ ). The output is 12, which is the value of the variable x. More about variables and data types will be explained in the next chapter of this lesson.
Python scripts
python script_name.py
If you have Linux OS, your program in script must begin with this line of code:
#!/usr/bin/python3
After that, you just write your code. When you want to run your script, in the Linux terminal run this command (make sure the script file has the executable flag):
./script_name.py
Keywords
Keywords are words that cannot be used for naming variables. They are reserved for a special use. Some keywords are used in loops (for, while, etc.), others are used in logical operations (True, False, and, etc.) and the list goes on. Here is a table with all keywords in Python:
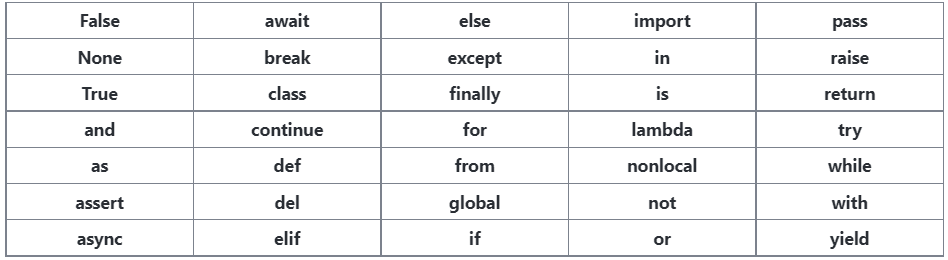
Here’s an example of correct use of a keyword:
>>> x = 8 and 15
>>> print(x)
15
We used keyword and for logical and operation between 8 and 15 to set a value for variable x, which is 15.
Here’s an example of incorrect use of a keyword:
>>> import = 'Jordan'
SyntaxError: Can't assign to keyword
We tried to use the keyword import as a name for the variable and set its value to ‘Jordan’ (string). As we can see, the program reports an error.
Comments
>>> x = 7 #definition of variable x
>>> y = 3.14 #definition of variable y
Docstrings are comments that can spread in multiple lines. They are written inside triple quotation marks (“”” “”” or ”’ ”’) and usually are used for documentation.
>>> y = 9
"""
variable
y
with
value
9
"""
Now that we’ve learnt about comments, we will use them for the most of explanations in future examples.
Table of contents
- Basic Data Structures
- Functions
- Collections
- Exceptions
- Input & Output
- RegEx & PRNG
- Classes And Objects
- Popular Libraries
- Additional Problems