Sets
Set definition
Sets are objects that represent collections of immutable objects (a list cannot be an element of a set). They are unordered collections, which means that the order of element insertion into set doesn’t matter and order of printing isn’t necessarily the same as the order of elements during the set’s creation. Elements of the set cannot be accessed by indexing.
Sets are created by assignment using { }, inside of which we define its elements (which are separated with , ). Sets can also be created with the command (constructor) set(), where the argument can be a string or a list. When creating a set, duplicates ( elements with the same value ) are ignored. An empty set can be created with the statement A = set(), but not with A = {} (this represents a different data structure).
>>> rgb = {"red", "green", "blue"} #creating a set by assigning values
>>> print(rgb)
{'red', 'blue', 'green'}
>>> A = set("waterfall") #creating a set using the constructor with character elements
>>> print(set)
{'l', 't', 'f', 'r', 'w', 'a', 'e'}
>>> B = set(['5', '6', '6', '7', '7', '7', '8', '8', '9', '10']) """creating a set using
the constructor with list elements"""
>>> print(grades)
{'8', '6', '10', '5', '9', '7'}
Iteration and membership
We can iterate through a set using a for-loop. Membership to a set can be tested with the membership operators in or not in, which return True if an element belongs to the set, otherwise they return False.
>>> set = {'elk', 'wolf', 'bear', 'fox', 'moose'}
>>> for i in set:
if len(i) == 3:
continue
print(i)
bear
moose
wolf
Attribute commands and operators for working with sets
In this table are attribute commands and operators which suit them, that can make our job easier while working with sets:
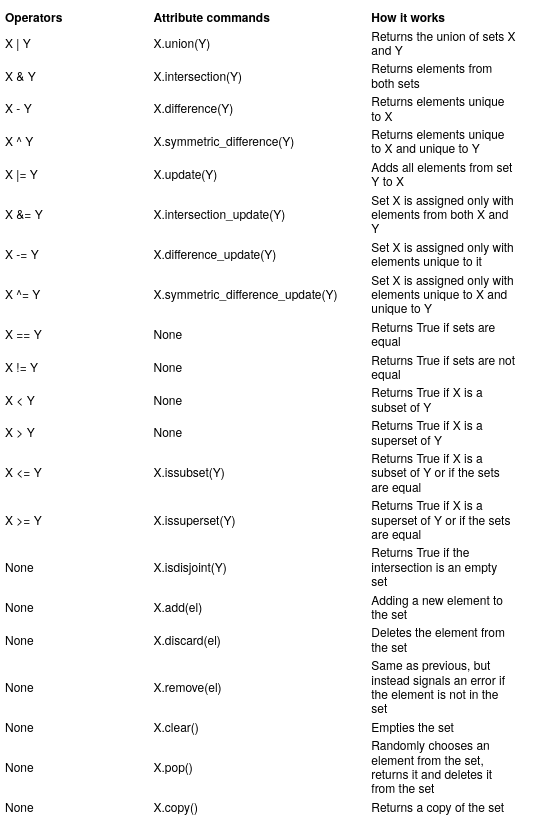
Here are a few examples:
>>> X = {1, 2, 3, 4, 5}
>>> Y = {3, 4, 5, 6, 7}
>>> print(X|Y)
{1, 2, 3, 4, 5, 6, 7}
>>> print(X&Y)
{3, 4, 5}
>>> print(X-Y, Y-X, X^Y)
{1, 2} {6, 7} {1, 2, 6, 7}
>>> X.add(0)
>>> print(X)
{0, 1, 2, 3, 4, 5}
>>> Y.remove(7)
>>> print(Y)
{3, 4, 5, 6}
Table of contents
- Functions
- Collections
- Exceptions
- Input & Output
- RegEx & PRNG
- Classes And Objects
- Popular Libraries
- Additional Problems